Python List, Tuple and Dictionary
Python List
List in python is implemented to store the sequence of various type of data. However, python contains six data types that are capable to store the sequences but the most common and reliable type is list.
A list can be defined as a collection of values or items of different types. The items in the list are separated with the comma (,) and enclosed with the square brackets [].
A list can be defined as follows.
If we try to print the type of L1, L2, and L3 then it will come out to be a list.
Lets consider a proper example to define a list and printing its values.
Output:
printing employee data... Name : John, ID: 102, Country: USA printing departments... Department 1: Name: CS, ID: 11 Department 2: Name: IT, ID: 11 HOD Details .... CS HOD Name: Mr. Holding, Id: 10 IT HOD Name: Mr. Bewon, Id: 11 <class 'list'> <class 'list'> <class 'list'> <class 'list'> <class 'list'>
List indexing and splitting
The indexing are processed in the same way as it happens with the strings. The elements of the list can be accessed by using the slice operator [].
The index starts from 0 and goes to length - 1. The first element of the list is stored at the 0th index, the second element of the list is stored at the 1st index, and so on.
Consider the following example.
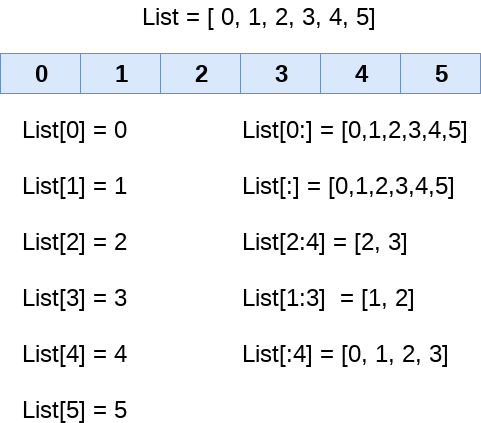
Unlike other languages, python provides us the flexibility to use the negative indexing also. The negative indices are counted from the right. The last element (right most) of the list has the index -1, its adjacent left element is present at the index -2 and so on until the left most element is encountered.

Updating List values
Lists are the most versatile data structures in python since they are immutable and their values can be updated by using the slice and assignment operator.
Python also provide us the append() method which can be used to add values to the string.
Consider the following example to update the values inside the list.
Output:
[1, 2, 3, 4, 5, 6] [1, 2, 10, 4, 5, 6] [1, 89, 78, 4, 5, 6]
The list elements can also be deleted by using the del keyword. Python also provides us the remove() method if we do not know which element is to be deleted from the list.
Consider the following example to delete the list elements.
Output:
[0, 1, 2, 3, 4] [1, 2, 3, 4] [1, 2, 3]
Python List Built-in functions
Python provides the following built-in functions which can be used with the lists.
SN | Function | Description |
---|---|---|
1 | cmp(list1, list2) | It compares the elements of both the lists. |
2 | len(list) | It is used to calculate the length of the list. |
3 | max(list) | It returns the maximum element of the list. |
4 | min(list) | It returns the minimum element of the list. |
5 | list(seq) | It converts any sequence to the list. |
Python List built-in methods
SN | Function | Description |
---|---|---|
1 | list.append(obj) | The element represented by the object obj is added to the list. |
2 | list.clear() | It removes all the elements from the list. |
3 | List.copy() | It returns a shallow copy of the list. |
4 | list.count(obj) | It returns the number of occurrences of the specified object in the list. |
5 | list.extend(seq) | The sequence represented by the object seq is extended to the list. |
6 | list.index(obj) | It returns the lowest index in the list that object appears. |
7 | list.insert(index, obj) | The object is inserted into the list at the specified index. |
8 | list.pop(obj=list[-1]) | It removes and returns the last object of the list. |
9 | list.remove(obj) | It removes the specified object from the list. |
10 | list.reverse() | It reverses the list. |
11 | list.sort([func]) | It sorts the list by using the specified compare function if given. |
Python Tuple
Python Tuple is used to store the sequence of immutable python objects. Tuple is similar to lists since the value of the items stored in the list can be changed whereas the tuple is immutable and the value of the items stored in the tuple can not be changed.
A tuple can be written as the collection of comma-separated values enclosed with the small brackets. A tuple can be defined as follows.
Example
Output:
(10, 20, 30, 40, 50, 60) tuple1[0] = 10 tuple1[0] = 20 tuple1[0] = 30 tuple1[0] = 40 tuple1[0] = 50 tuple1[0] = 60
Tuple indexing and splitting
The indexing and slicing in tuple are similar to lists. The indexing in the tuple starts from 0 and goes to length(tuple) - 1.
The items in the tuple can be accessed by using the slice operator. Python also allows us to use the colon operator to access multiple items in the tuple.
Consider the following image to understand the indexing and slicing in detail.
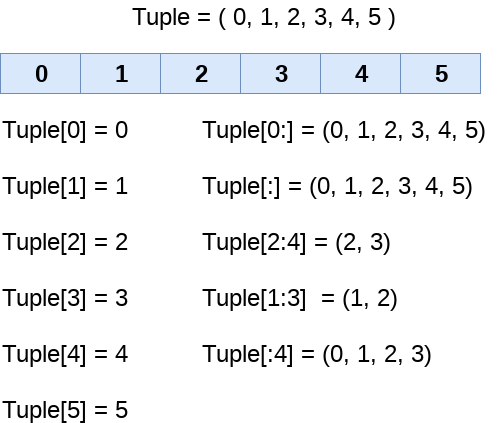
Unlike lists, the tuple items can not be deleted by using the del keyword as tuples are immutable. To delete an entire tuple, we can use the del keyword with the tuple name.
Consider the following example.
Output:
(1, 2, 3, 4, 5, 6) Traceback (most recent call last): File "tuple.py", line 4, in <module> print(tuple1) NameError: name 'tuple1' is not defined
Like lists, the tuple elements can be accessed in both the directions. The right most element (last) of the tuple can be accessed by using the index -1. The elements from left to right are traversed using the negative indexing.
Consider the following example.
Output:
5 2
Python Tuple inbuilt functions
SN | Function | Description |
---|---|---|
1 | cmp(tuple1, tuple2) | It compares two tuples and returns true if tuple1 is greater than tuple2 otherwise false. |
2 | len(tuple) | It calculates the length of the tuple. |
3 | max(tuple) | It returns the maximum element of the tuple. |
4 | min(tuple) | It returns the minimum element of the tuple. |
5 | tuple(seq) | It converts the specified sequence to the tuple. |
Python Dictionary
Dictionary is used to implement the key-value pair in python. The dictionary is the data type in python which can simulate the real-life data arrangement where some specific value exists for some particular key.
In other words, we can say that a dictionary is the collection of key-value pairs where the value can be any python object whereas the keys are the immutable python object, i.e., Numbers, string or tuple.
Dictionary simulates Java hash-map in python.
Creating the dictionary
The dictionary can be created by using multiple key-value pairs enclosed with the small brackets () and separated by the colon (:). The collections of the key-value pairs are enclosed within the curly braces {}.
The syntax to define the dictionary is given below.
In the above dictionary Dict, The keys Name, and Age are the string that is an immutable object.
Let's see an example to create a dictionary and printing its content.
Output
<class 'dict'> printing Employee data .... {'Age': 29, 'salary': 25000, 'Name': 'John', 'Company': 'GOOGLE'}
Accessing the dictionary values
We have discussed how the data can be accessed in the list and tuple by using the indexing.
However, the values can be accessed in the dictionary by using the keys as keys are unique in the dictionary.
The dictionary values can be accessed in the following way.
Output:
<class 'dict'> printing Employee data .... Name : John Age : 29 Salary : 25000 Company : GOOGLE
Python provides us with an alternative to use the get() method to access the dictionary values. It would give the same result as given by the indexing.
Updating dictionary values
The dictionary is a mutable data type, and its values can be updated by using the specific keys.
Let's see an example to update the dictionary values.
Output:
<class 'dict'> printing Employee data .... {'Name': 'John', 'salary': 25000, 'Company': 'GOOGLE', 'Age': 29} Enter the details of the new employee.... Name: David Age: 19 Salary: 8900 Company:JTP printing the new data {'Name': 'David', 'salary': 8900, 'Company': 'JTP', 'Age': 19}
Deleting elements using del keyword
The items of the dictionary can be deleted by using the del keyword as given below.
Output:
<class 'dict'> printing Employee data .... {'Age': 29, 'Company': 'GOOGLE', 'Name': 'John', 'salary': 25000} Deleting some of the employee data printing the modified information {'Age': 29, 'salary': 25000} Deleting the dictionary: Employee Lets try to print it again Traceback (most recent call last): File "list.py", line 13, in <module> print(Employee) NameError: name 'Employee' is not defined
Iterating Dictionary
A dictionary can be iterated using the for loop as given below.
Example 1
# for loop to print all the keys of a dictionary
Output:
Name Company salary Age
Built-in Dictionary functions
The built-in python dictionary methods along with the description are given below.
SN | Function | Description |
---|---|---|
1 | cmp(dict1, dict2) | It compares the items of both the dictionary and returns true if the first dictionary values are greater than the second dictionary, otherwise it returns false. |
2 | len(dict) | It is used to calculate the length of the dictionary. |
3 | str(dict) | It converts the dictionary into the printable string representation. |
4 | type(variable) | It is used to print the type of the passed variable. |
Built-in Dictionary methods
The built-in python dictionary methods along with the description are given below.
SN | Method | Description |
---|---|---|
1 | dic.clear() | It is used to delete all the items of the dictionary. |
2 | dict.copy() | It returns a shallow copy of the dictionary. |
3 | dict.fromkeys(iterable, value = None, /) | Create a new dictionary from the iterable with the values equal to value. |
4 | dict.get(key, default = "None") | It is used to get the value specified for the passed key. |
5 | dict.has_key(key) | It returns true if the dictionary contains the specified key. |
6 | dict.items() | It returns all the key-value pairs as a tuple. |
7 | dict.keys() | It returns all the keys of the dictionary. |
8 | dict.setdefault(key,default= "None") | It is used to set the key to the default value if the key is not specified in the dictionary |
9 | dict.update(dict2) | It updates the dictionary by adding the key-value pair of dict2 to this dictionary. |
10 | dict.values() | It returns all the values of the dictionary. |
11 | len() | |
12 | popItem() | |
13 | pop() | |
14 | count() | |
15 | index() |
Comments
Post a Comment